OOPS Concepts:
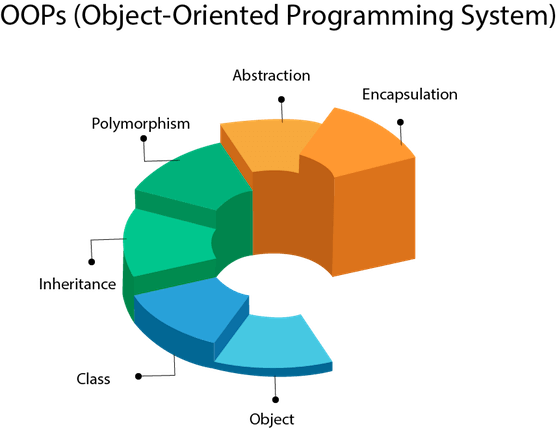
Object
Any entity that has state and behaviour is known as an object.
For example, a chair, pen, table, keyboard, bike, etc. It can be physical or logical.
An Object can be defined as an instance of a class
An object has three characteristics:
- State: represents the data (value) of an object.
- Behavior: represents the behavior (functionality) of an object such as deposit, withdraw, etc.
- Identity: An object identity is typically implemented via a unique ID. The value of the ID is not visible to the external user. However, it is used internally by the JVM to identify each object uniquely.
Example: A dog is an object because it has states like color, name, breed, etc. as well as behaviors like wagging the tail, barking, eating, etc.
Class
Collection of objects is called class. It is a logical entity.
A class can also be defined as a blueprint from which you can create an individual object.
Inheritance
When one object acquires all the properties and behaviors of a parent object, it is known as inheritance. It provides code reusability. It is used to achieve runtime polymorphism.
The idea behind inheritance in Java is that you can create new classes that are built upon existing classes. When you inherit from an existing class, you can reuse methods and fields of the parent class. Moreover, you can add new methods and fields in your current class also.
Inheritance represents the IS-A relationship which is also known as a parent-child relationship.
Why use inheritance in java
The idea behind inheritance in Java is that you can create new classes that are built upon existing classes. When you inherit from an existing class, you can reuse methods and fields of the parent class. Moreover, you can add new methods and fields in your current class also.
Inheritance represents the IS-A relationship which is also known as a parent-child relationship.
Why use inheritance in java
- For Method Overriding (so runtime polymorphism can be achieved).
- For Code Reusability.
The syntax of Java Inheritance
- class Subclass-name extends Superclass-name
- {
- //methods and fields
- }
The extends keyword indicates that you are making a new class that derives from an existing class. The meaning of "extends" is to increase the functionality.
In the terminology of Java, a class which is inherited is called a parent or superclass, and the new class is called child or subclass.
Java Inheritance Example
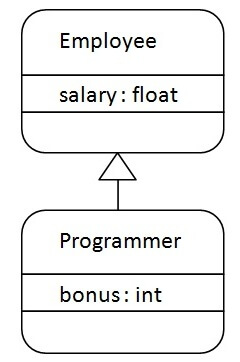
As displayed in the above figure, Programmer is the subclass and Employee is the superclass. The relationship between the two classes is Programmer IS-A Employee. It means that Programmer is a type of Employee.
- class Employee{
- float salary=40000;
- }
- class Programmer extends Employee{
- int bonus=10000;
- public static void main(String args[]){
- Programmer p=new Programmer();
- System.out.println("Programmer salary is:"+p.salary);
- System.out.println("Bonus of Programmer is:"+p.bonus);
- }
- }
O/P
Programmer salary is:40000.0 Bonus of programmer is:10000
In the above example, Programmer object can access the field of own class as well as of Employee class i.e. code reusability.
Types of inheritance in javaOn the basis of class, there can be three types of inheritance in java: single, multilevel and hierarchical.In java programming, multiple and hybrid inheritance is supported through interface only. We will learn about interfaces later.
Note: Multiple inheritance is not supported in Java through class.
When one class inherits multiple classes, it is known as multiple inheritance. For Example:
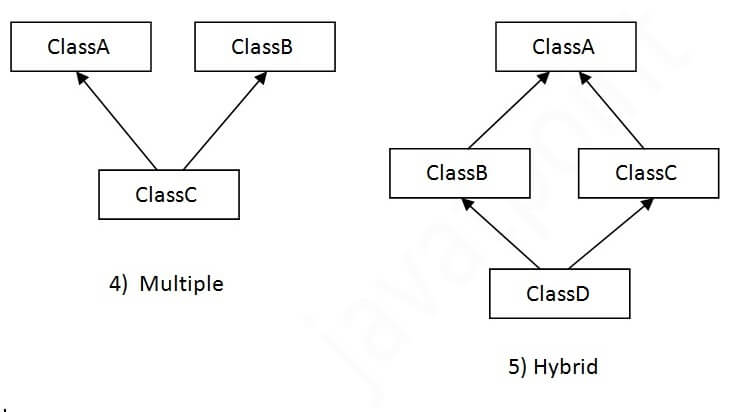
When a class inherits another class, it is known as a single inheritance. In the example given below, Dog class inherits the Animal class, so there is the single inheritance.
File: TestInheritance.java
Multilevel Inheritance Example
When there is a chain of inheritance, it is known as multilevel inheritance. As you can see in the example given below, BabyDog class inherits the Dog class which again inherits the Animal class, so there is a multilevel inheritance.
- class Animal{
- void eat(){System.out.println("eating...");}
- }
- class Dog extends Animal{
- void bark(){System.out.println("barking...");}
- }
- class BabyDog extends Dog{
- void weep(){System.out.println("weeping...");}
- }
- class TestInheritance2{
- public static void main(String args[]){
- BabyDog d=new BabyDog();
- d.weep();
- d.bark();
- d.eat();
- }}
Output:
weeping... barking... eating...
Hierarchical Inheritance Example
When two or more classes inherits a single class, it is known as hierarchical inheritance. In the example given below, Dog and Cat classes inherits the Animal class, so there is hierarchical inheritance.
- class Animal{
- void eat(){System.out.println("eating...");}
- }
- class Dog extends Animal{
- void bark(){System.out.println("barking...");}
- }
- class Cat extends Animal{
- void meow(){System.out.println("meowing...");}
- }
- class TestInheritance3{
- public static void main(String args[]){
- Cat c=new Cat();
- c.meow();
- c.eat();
- //c.bark();//C.T.Error
- }}
O/Pmeowing... eating...Q) Why multiple inheritance is not supported in java?
To reduce the complexity and simplify the language, multiple inheritance is not supported in java.Consider a scenario where A, B, and C are three classes. The C class inherits A and B classes. If A and B classes have the same method and you call it from child class object, there will be ambiguity to call the method of A or B class.Since compile-time errors are better than runtime errors, Java renders compile-time error if you inherit 2 classes. So whether you have same method or different, there will be compile time error.
- class A{
- void msg(){System.out.println("Hello");}
- }
- class B{
- void msg(){System.out.println("Welcome");}
- }
- class C extends A,B{//suppose if it were
- public static void main(String args[]){
- C obj=new C();
- obj.msg();//Now which msg() method would be invoked?
- }
- }
O/PCompile Time Error
Abstract class in Java
Hiding internal details and showing functionality is known as abstraction. For example phone call, we don't know the internal processing.
In Java, we use abstract class and interface to achieve abstraction.
Another way, it shows only essential things to the user and hides the internal details, for example, sending SMS where you type the text and send the message. You don't know the internal processing about the message delivery.
Abstraction lets you focus on what the object does instead of how it does it.
Ways to achieve Abstraction
There are two ways to achieve abstraction in java- Abstract class (0 to 100%)
- Interface (100%)
Abstract class in Java
A class which is declared as abstract is known as an abstract class. It can have abstract and non-abstract methods. It needs to be extended and its method implemented. It cannot be instantiated.
Points to Remember
- An abstract class must be declared with an abstract keyword.
- It can have abstract and non-abstract methods.
- It cannot be instantiated.
- It can have constructors and static methods also.
- It can have final methods which will force the subclass not to change the body of the method.

Example of abstract class
abstract class A{}
Abstract Method in Java
A method which is declared as abstract and does not have implementation is known as an abstract method.
Example of abstract method
abstract void printStatus();//no method body and abstract
Example of Abstract class that has an abstract method
- abstract class Bike{
- abstract void run();
- }
- class Honda4 extends Bike{
- void run(){System.out.println("running safely");}
- public static void main(String args[]){
- Bike obj = new Honda4();
- obj.run();
- }
- }
O/P:
running safely
Understanding the real scenario of Abstract class
- abstract class Shape{
- abstract void draw();
- }
- //In real scenario, implementation is provided by others i.e. unknown by end user
- class Rectangle extends Shape{
- void draw(){System.out.println("drawing rectangle");}
- }
- class Circle1 extends Shape{
- void draw(){System.out.println("drawing circle");}
- }
- //In real scenario, method is called by programmer or user
- class TestAbstraction1{
- public static void main(String args[]){
- Shape s=new Circle1();//In a real scenario, object is provided through method, e.g., getShape() method
- s.draw();
- }
- }
drawing circleAnother example of Abstract class in java
- abstract class Bank{
- abstract int getRateOfInterest();
- }
- class SBI extends Bank{
- int getRateOfInterest(){return 7;}
- }
- class PNB extends Bank{
- int getRateOfInterest(){return 8;}
- }
- class TestBank{
- public static void main(String args[]){
- Bank b;
- b=new SBI();
- System.out.println("Rate of Interest is: "+b.getRateOfInterest()+" %");
- b=new PNB();
- System.out.println("Rate of Interest is: "+b.getRateOfInterest()+" %");
- }}
Rate of Interest is: 7 % Rate of Interest is: 8 %Abstract class having constructor, data member and methods
An abstract class can have a data member, abstract method, method body (non-abstract method), constructor, and even main() method.
- //Example of an abstract class that has abstract and non-abstract methods
- abstract class Bike{
- Bike(){System.out.println("bike is created");}
- abstract void run();
- void changeGear(){System.out.println("gear changed");}
- }
- //Creating a Child class which inherits Abstract class
- class Honda extends Bike{
- void run(){System.out.println("running safely..");}
- }
- //Creating a Test class which calls abstract and non-abstract methods
- class TestAbstraction2{
- public static void main(String args[]){
- Bike obj = new Honda();
- obj.run();
- obj.changeGear();
- }
- }
bike is created running safely.. gear changedRule: If there is an abstract method in a class, that class must be abstract.
- class Bike12{
- abstract void run();
- }
compile time errorRule: If you are extending an abstract class that has an abstract method, you must either provide the implementation of the method or make this class abstract.
Another real scenario of abstract class
The abstract class can also be used to provide some implementation of the interface. In such case, the end user may not be forced to override all the methods of the interface.
- interface A{
- void a();
- void b();
- void c();
- void d();
- }
-
- abstract class B implements A{
- public void c(){System.out.println("I am c");}
- }
-
- class M extends B{
- public void a(){System.out.println("I am a");}
- public void b(){System.out.println("I am b");}
- public void d(){System.out.println("I am d");}
- }
-
- class Test5{
- public static void main(String args[]){
- A a=new M();
- a.a();
- a.b();
- a.c();
- a.d();
- }}
Output:I am a
I am b
I am c
I am d
Output:I am a I am b I am c I am d
Encapsulation
Encapsulation in Java is a process of wrapping code and data together into a single unit, for example, a capsule which is mixed of several medicines.
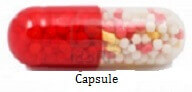
We can create a fully encapsulated class in Java by making all the data members of the class private. Now we can use setter and getter methods to set and get the data in it.
The Java Bean class is the example of a fully encapsulated class.
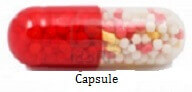
We can create a fully encapsulated class in Java by making all the data members of the class private. Now we can use setter and getter methods to set and get the data in it.
The Java Bean class is the example of a fully encapsulated class.
By providing only a setter or getter method, you can make the class read-only or write-only. In other words, you can skip the getter or setter methods.
It provides you the control over the data.
It is a way to achieve data hiding in Java because other class will not be able to access the data through the private data members.
The encapsulate class is easy to test. So, it is better for unit testing.
The standard IDE's are providing the facility to generate the getters and setters. So, it is easy and fast to create an encapsulated class in Java.
Simple Example of Encapsulation in Java
- //A Java class which is a fully encapsulated class.
- //It has a private data member and getter and setter methods.
- package com.javatpoint;
- public class Student{
- //private data member
- private String name;
- //getter method for name
- public String getName(){
- return name;
- }
- //setter method for name
- public void setName(String name){
- this.name=name
- }
- }
File: Test.java
- //A Java class to test the encapsulated class.
- package com.javatpoint;
- class Test{
- public static void main(String[] args){
- //creating instance of the encapsulated class
- Student s=new Student();
- //setting value in the name member
- s.setName("vijay");
- //getting value of the name member
- System.out.println(s.getName());
- }
- }
Output: vijay
Polymorphism in Java
Polymorphism in Java is a concept by which we can perform a single action in different ways. polymorphism means many forms. For example: to convince the customer differently, to draw something, for example, shape, triangle, rectangle, etc.
In Java, we use method overloading and method overriding to achieve polymorphism.
Another example can be to speak something; for example, a cat speaks meow, dog barks woof, etc.
- class Animal{
- void eat(){System.out.println("eating...");}
- }
- class Dog extends Animal{
- void bark(){System.out.println("barking...");}
- }
- class TestInheritance{
- public static void main(String args[]){
- Dog d=new Dog();
- d.bark();
- d.eat();
- }}
O/P
barking... eating...
There are two types of polymorphism in Java: compile-time polymorphism and runtime polymorphism. We can perform polymorphism in java by method overloading and method overriding.
If you overload a static method in Java, it is the example of compile time polymorphism. Here, we will focus on runtime polymorphism in java.
Runtime Polymorphism in Java
Runtime polymorphism or Dynamic Method Dispatch is a process in which a call to an overridden method is resolved at runtime rather than compile-time.
In this process, an overridden method is called through the reference variable of a superclass. The determination of the method to be called is based on the object being referred to by the reference variable.
Let's first understand the upcasting before Runtime Polymorphism.
Upcasting
If the reference variable of Parent class refers to the object of Child class, it is known as upcasting. For example:
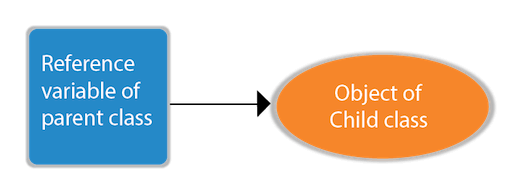
- class A{}
- class B extends A{}
A a=new B();//upcasting
For upcasting, we can use the reference variable of class type or an interface type. For Example:
- interface I{}
- class A{}
- class B extends A implements I{}
Here, the relationship of B class would be:
B IS-A A B IS-A I B IS-A Object
Since Object is the root class of all classes in Java, so we can write B IS-A Object.
Example of Java Runtime Polymorphism
In this example, we are creating two classes Bike and Splendor. Splendor class extends Bike class and overrides its run() method. We are calling the run method by the reference variable of Parent class. Since it refers to the subclass object and subclass method overrides the Parent class method, the subclass method is invoked at runtime.
Since method invocation is determined by the JVM not compiler, it is known as runtime polymorphism.
- class Bike{
- void run(){System.out.println("running");}
- }
- class Splendor extends Bike{
- void run(){System.out.println("running safely with 60km");}
- public static void main(String args[]){
- Bike b = new Splendor();//upcasting
- b.run();
- }
- }
Output: running safely with 60km.
Java Runtime Polymorphism Example: Bank
Consider a scenario where Bank is a class that provides a method to get the rate of interest. However, the rate of interest may differ according to banks. For example, SBI, ICICI, and AXIS banks are providing 8.4%, 7.3%, and 9.7% rate of interest.

- class Bank{
- float getRateOfInterest(){return 0;}
- }
- class SBI extends Bank{
- float getRateOfInterest(){return 8.4f;}
- }
- class ICICI extends Bank{
- float getRateOfInterest(){return 7.3f;}
- }
- class AXIS extends Bank{
- float getRateOfInterest(){return 9.7f;}
- }
- class TestPolymorphism{
- public static void main(String args[]){
- Bank b;
- b=new SBI();
- System.out.println("SBI Rate of Interest: "+b.getRateOfInterest());
- b=new ICICI();
- System.out.println("ICICI Rate of Interest: "+b.getRateOfInterest());
- b=new AXIS();
- System.out.println("AXIS Rate of Interest: "+b.getRateOfInterest());
- }
- }
Output:
SBI Rate of Interest: 8.4 ICICI Rate of Interest: 7.3 AXIS Rate of Interest: 9.7
No comments:
Post a Comment